================
by Jawad Haider
02 - PyTorch Basics Exercises SOLUTION¶
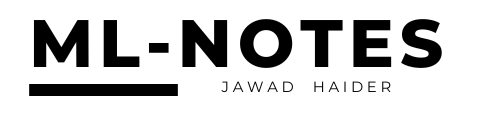
- 1 PyTorch Basics Exercises - SOLUTIONS
- 1.0.1 1. Perform standard imports
- 1.0.2 2. Set the random seed for NumPy and PyTorch both to “42”
- 1.0.3 3. Create a NumPy array called “arr” that contains 6 random integers between 0 (inclusive) and 5 (exclusive)
- 1.0.4 4. Create a tensor “x” from the array above
- 1.0.5 5. Change the dtype of x from ‘int32’ to ‘int64’
- 1.0.6 6. Reshape x into a 3x2 tensor
- 1.0.7 7. Return the right-hand column of tensor x
- 1.0.8 8. Without changing x, return a tensor of square values of x
- 1.0.9 9. Create a tensor “y” with the same number of elements as x, that can be matrix-multiplied with x
- 1.0.10 10. Find the matrix product of x and y
- 1.1 Great job!
PyTorch Basics Exercises - SOLUTIONS¶
For these exercises we’ll create a tensor and perform several operations on it.
IMPORTANT NOTE! Make sure you don’t run the cells
directly above the example output shown,
otherwise you will end up writing over the example output!
otherwise you will end up writing over the example output!
1. Perform standard imports¶
Import torch and NumPy
2. Set the random seed for NumPy and PyTorch both to “42”¶
This allows us to share the same “random” results.
# CODE HERE
np.random.seed(42)
torch.manual_seed(42); # the semicolon suppresses the jupyter output line
3. Create a NumPy array called “arr” that contains 6 random integers between 0 (inclusive) and 5 (exclusive)¶
[3 4 2 4 4 1]
4. Create a tensor “x” from the array above¶
tensor([3, 4, 2, 4, 4, 1], dtype=torch.int32)
5. Change the dtype of x from ‘int32’ to ‘int64’¶
Note: ‘int64’ is also called ‘LongTensor’
torch.LongTensor
6. Reshape x into a 3x2 tensor¶
There are several ways to do this.
tensor([[3, 4],
[2, 4],
[4, 1]])
7. Return the right-hand column of tensor x¶
tensor([[4],
[4],
[1]])
8. Without changing x, return a tensor of square values of x¶
There are several ways to do this.
# DON'T WRITE HERE
print(x*x)
# print(x**2)
# print(x.mul(x))
# print(x.pow(2))
# print(torch.mul(x,x))
tensor([[ 9, 16],
[ 4, 16],
[16, 1]])
9. Create a tensor “y” with the same number of elements as x, that can be matrix-multiplied with x¶
Use PyTorch directly (not NumPy) to create a tensor of random integers
between 0 (inclusive) and 5 (exclusive).
Think about what shape it
should have to permit matrix multiplication.
tensor([[2, 2, 1],
[4, 1, 0]])
10. Find the matrix product of x and y¶
tensor([[22, 10, 3],
[20, 8, 2],
[12, 9, 4]])