================ by Jawad Haider
01 - Series¶
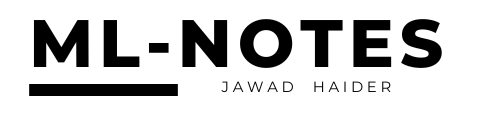
Series¶
The first main data type we will learn about for pandas is the Series data type. Let’s import Pandas and explore the Series object.
A Series is very similar to a NumPy array (in fact it is built on top of the NumPy array object). What differentiates the NumPy array from a Series, is that a Series can have axis labels, meaning it can be indexed by a label, instead of just a number location. It also doesn’t need to hold numeric data, it can hold any arbitrary Python Object.
Let’s explore this concept through some examples:
Creating a Series¶
You can convert a list,numpy array, or dictionary to a Series:
Using Lists¶
0 10
1 20
2 30
dtype: int64
a 10
b 20
c 30
dtype: int64
a 10
b 20
c 30
dtype: int64
Using NumPy Arrays¶
0 10
1 20
2 30
dtype: int64
a 10
b 20
c 30
dtype: int64
Using Dictionaries¶
a 10
b 20
c 30
dtype: int64
Data in a Series¶
A pandas Series can hold a variety of object types:
0 a
1 b
2 c
dtype: object
0 <built-in function sum>
1 <built-in function print>
2 <built-in function len>
dtype: object
Using an Index¶
The key to using a Series is understanding its index. Pandas makes use of these index names or numbers by allowing for fast look ups of information (works like a hash table or dictionary).
Let’s see some examples of how to grab information from a Series. Let us create two sereis, ser1 and ser2:
USA 1
Germany 2
USSR 3
Japan 4
dtype: int64
USA 1
Germany 2
Italy 5
Japan 4
dtype: int64
1
Operations are then also done based off of index:
Germany 4.0
Italy NaN
Japan 8.0
USA 2.0
USSR NaN
dtype: float64
Great Job! Thats the end of this part.¶
Don't forget to give a star on github and follow for more curated Computer Science, Machine Learning materials